I wanna perform a function like this. When you save a record, I need the primary key of that record to be auto-generated (X001). Say, when you create a new record and save it, it should be saved like X002 automatically.
So to test this before I implement that on this project I'm working on, I created this little program.
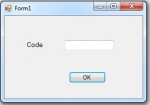
All its supposed to do is, when you click on the OK button it shows a code that is fetched from a database table. (X001). Then when you click on it ober and over again. The number should increase. X002, X003, X004... so on.
Here's my code
But when I run it, it throws this conversion error.
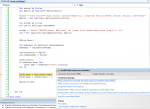
I know its pretty straight forward so I tried to correct it with what I know but it wasn't successful so I searched the net for solutions. And tried some of them.
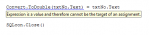
But nothing worked.
I'd be very grateful if someone would help me with this. I know this is be very simple but forgive me since I'm new to VB.NET.
Thanks very much.
I.J
So to test this before I implement that on this project I'm working on, I created this little program.
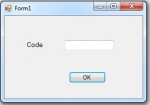
All its supposed to do is, when you click on the OK button it shows a code that is fetched from a database table. (X001). Then when you click on it ober and over again. The number should increase. X002, X003, X004... so on.
Here's my code
VB.NET:
Imports System.Data.SqlClient
Public Class Form1
Private Sub btnOK_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnOK.Click
Dim strcon As String
Dim SQLcon As SqlClient.SqlConnection
strcon = "data source=winux; initial catalog=temperory; integrated security=SSPI; persist security info=false"
SQLcon = New SqlClient.SqlConnection(strcon)
Dim strsql As String
Dim com As SqlClient.SqlCommand
strsql = "select CONVERT(nchar, MAX(code)) as [autocode] from CodeAutoGen where form_id = '05'"
com = New SqlClient.SqlCommand(strsql, SQLcon)
SQLcon.Open()
Dim myReader As SqlClient.SqlDataReader
myReader = com.ExecuteReader
com.Connection = SQLcon
com.CommandText = strsql
txtNo.Clear()
If myReader.Read Then
txtNo.Text = myReader("code").ToString
End If
'txtNo.Text = CDbl(txtNo.Text)
'txtNo.Text = (txtNo.Text) + 1
SQLcon.Close()
End Sub
End Class
But when I run it, it throws this conversion error.
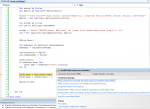
I know its pretty straight forward so I tried to correct it with what I know but it wasn't successful so I searched the net for solutions. And tried some of them.
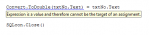
But nothing worked.
I'd be very grateful if someone would help me with this. I know this is be very simple but forgive me since I'm new to VB.NET.
Thanks very much.
I.J