Hi,
I made a simple Form1, with Text.Box1 (starting value=1) and Text.Box2 (starting value = 100000), as shown below :
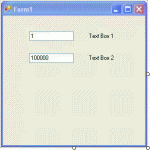
I wanted that, when the Form loads,value of Text.Box1 keeps on increasing by 2 and value of Text.Box2 keeps on decreasing by 3. I wanted to achieve this by multi-threading.
Following is the code I wrote, which definitely has some problem with it.
*************************************************************
Imports System.Threading.Thread
Public Delegate Sub funcHolder(ByVal diff1234 As Integer)
Public Class Form1
Dim i As Integer
Dim j As Integer
'-------------------------------------------------------------
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim upd12Holder As funcHolder
Dim upd34Holder As funcHolder
upd12Holder = AddressOf upd12
upd34Holder = AddressOf upd34
Dim t12 As New System.Threading.Thread(AddressOf upd12)
t12.Start(2)
Dim t34 As New System.Threading.Thread(AddressOf upd12)
t12.Start(3)
End Sub
'-------------------------------------------------------------
Public Sub upd12(ByVal diff12 As Integer)
While (1)
TextBox1.Text = TextBox1.Text + diff12
For i = 1 To 10000 'delay loop
Next i
End While
End Sub
'-------------------------------------------------------------
Public Sub upd34(ByVal diff34 As Integer)
While (1)
TextBox2.Text = TextBox2.Text - diff34
For j = 1 To 10000 'delay loop
Next j
End While
End Sub
'-------------------------------------------------------------
End Class
*************************************************************
It would be very helpful if you could help me identify and correct the problem.
Thank you all.
I made a simple Form1, with Text.Box1 (starting value=1) and Text.Box2 (starting value = 100000), as shown below :
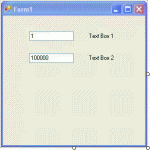
I wanted that, when the Form loads,value of Text.Box1 keeps on increasing by 2 and value of Text.Box2 keeps on decreasing by 3. I wanted to achieve this by multi-threading.
Following is the code I wrote, which definitely has some problem with it.
*************************************************************
Imports System.Threading.Thread
Public Delegate Sub funcHolder(ByVal diff1234 As Integer)
Public Class Form1
Dim i As Integer
Dim j As Integer
'-------------------------------------------------------------
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim upd12Holder As funcHolder
Dim upd34Holder As funcHolder
upd12Holder = AddressOf upd12
upd34Holder = AddressOf upd34
Dim t12 As New System.Threading.Thread(AddressOf upd12)
t12.Start(2)
Dim t34 As New System.Threading.Thread(AddressOf upd12)
t12.Start(3)
End Sub
'-------------------------------------------------------------
Public Sub upd12(ByVal diff12 As Integer)
While (1)
TextBox1.Text = TextBox1.Text + diff12
For i = 1 To 10000 'delay loop
Next i
End While
End Sub
'-------------------------------------------------------------
Public Sub upd34(ByVal diff34 As Integer)
While (1)
TextBox2.Text = TextBox2.Text - diff34
For j = 1 To 10000 'delay loop
Next j
End While
End Sub
'-------------------------------------------------------------
End Class
*************************************************************
It would be very helpful if you could help me identify and correct the problem.
Thank you all.