Superfluper
New member
- Joined
- Aug 2, 2014
- Messages
- 1
- Programming Experience
- 1-3
Hello people,
I need some help with my paint program, I want to save my edited picturebox into a jpeg file but when I try to do it I get an error.
I have read on another forum that i can't save my picturebox edited with brushes and that I need to make graphics instead but I don't know how to do it :s
Please help me out here
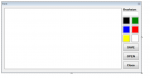
This is my code:
I need some help with my paint program, I want to save my edited picturebox into a jpeg file but when I try to do it I get an error.
I have read on another forum that i can't save my picturebox edited with brushes and that I need to make graphics instead but I don't know how to do it :s
Please help me out here

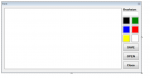
This is my code:
Public Class frmPaint Dim down = False Dim mybrush = Brushes.Black Dim BrushSize As Decimal Private Sub picDrawing_MouseDown(ByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles picDrawing.MouseDown down = True End Sub Private Sub picDrawing_MouseMove(ByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles picDrawing.MouseMove If txtBrushSize.Text = "" Then txtBrushSize.Text = 0 Else txtBrushSize.Text = txtBrushSize.Text End If If down = True Then picDrawing.CreateGraphics.FillEllipse(mybrush, e.X, e.Y, BrushSize, BrushSize) End If End Sub Private Sub picDrawing_MouseUp(ByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles picDrawing.MouseUp down = False End Sub Private Sub btnBlack_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnBlack.Click mybrush = Brushes.Black End Sub Private Sub btnGreen_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnGreen.Click mybrush = Brushes.Green End Sub Private Sub btnBlue_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnBlue.Click mybrush = Brushes.Blue End Sub Private Sub btnRed_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnRed.Click mybrush = Brushes.Red End Sub Private Sub btnYellow_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnYellow.Click mybrush = Brushes.Yellow End Sub Private Sub btnErase_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnErase.Click mybrush = Brushes.White End Sub Private Sub btnClose_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnClose.Click Me.Close() End Sub Private Sub txtBrushSize_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles txtBrushSize.TextChanged BrushSize = txtBrushSize.Text End Sub Private Sub btnSave_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnSave.Click SaveFileDialog1.ShowDialog() End Sub Private Sub btnOpen_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnOpen.Click OpenFileDialog1.ShowDialog() End Sub Private Sub OpenFileDialog1_FileOk(ByVal sender As System.Object, ByVal e As System.ComponentModel.CancelEventArgs) Handles OpenFileDialog1.FileOk picDrawing.ImageLocation = OpenFileDialog1.FileName End Sub Private Sub SaveFileDialog1_FileOk(ByVal sender As System.Object, ByVal e As System.ComponentModel.CancelEventArgs) Handles SaveFileDialog1.FileOk picDrawing.Image.Save(SaveFileDialog1.FileName + ".jpg", System.Drawing.Imaging.ImageFormat.Jpeg) End Sub Private Sub frmPaint_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load txtBrushSize.Text = 30 End Sub End Class
Last edited by a moderator: