stickysolutionz
New member
- Joined
- Jun 13, 2017
- Messages
- 3
- Programming Experience
- Beginner
I wrote a simple application to interface with Storj.io. Stroj is a decentralized cloud storage concept. It is driven by libstorj, a simple CLI. I wrote a gui to perform simple tasks like, list buckets, list files, upload download and delete. The code below is working 100%, that was until the other day, libstorj came out with a new binary, and this binary will not accept the method below. Let me focus where this is failing. with the old binary I could simply
MyProcess.StandardInput.WriteLine(InputTextBox.Text)
MyProcess.StandardInput.WriteLine(PWTextBox.Text)
MyProcess.StandardInput.Flush()
This would first launch a predetermined command defined in inputtextbox.text to console and then supply the password, which is the next prompt. So in reality line 1 would execute "storj.exe list-buckets" - this command would then ask for a password, the following line would satisfy that request "MyProcess.StandardInput.WriteLine(PWTextBox.Text)" This all went to sh1t with the new binary. Now what happens is, the console doesn't receive the password properly. basically it executes "storj.exe list-buckets" but the pw doesn't get entered, a return seems to be executed and then MyProcess.StandardInput.WriteLine(PWTextBox.Text) is displayed as a command itself rather than being inserted as the pw. Jesus, reading this back is confusing as hell, I hope someone can make sense of this.
My source, and a landing page on general use can be found at http://my.uselessinter.net/projects/storjgui/
If someone, can give me an idea on how else I can interact with cmd through windows forms that would be DOPE!!!
Thanks in advance
Heres my complete form1 source.
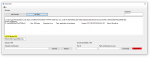
MyProcess.StandardInput.WriteLine(InputTextBox.Text)
MyProcess.StandardInput.WriteLine(PWTextBox.Text)
MyProcess.StandardInput.Flush()
This would first launch a predetermined command defined in inputtextbox.text to console and then supply the password, which is the next prompt. So in reality line 1 would execute "storj.exe list-buckets" - this command would then ask for a password, the following line would satisfy that request "MyProcess.StandardInput.WriteLine(PWTextBox.Text)" This all went to sh1t with the new binary. Now what happens is, the console doesn't receive the password properly. basically it executes "storj.exe list-buckets" but the pw doesn't get entered, a return seems to be executed and then MyProcess.StandardInput.WriteLine(PWTextBox.Text) is displayed as a command itself rather than being inserted as the pw. Jesus, reading this back is confusing as hell, I hope someone can make sense of this.
My source, and a landing page on general use can be found at http://my.uselessinter.net/projects/storjgui/
If someone, can give me an idea on how else I can interact with cmd through windows forms that would be DOPE!!!
Thanks in advance

Heres my complete form1 source.
Public Class Form1 Private WithEvents MyProcess As Process Private Delegate Sub AppendOutputTextDelegate(ByVal text As String) Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load Me.AcceptButton = ListBucketsButton MyProcess = New Process With MyProcess.StartInfo .FileName = "CMD.EXE" .UseShellExecute = False .CreateNoWindow = True .RedirectStandardInput = True .RedirectStandardOutput = True .RedirectStandardError = True End With MyProcess.Start() MyProcess.BeginErrorReadLine() MyProcess.BeginOutputReadLine() AppendOutputText("Process Started at: " & MyProcess.StartTime.ToString) End Sub Private Sub Form1_FormClosing(ByVal sender As Object, ByVal e As System.Windows.Forms.FormClosingEventArgs) Handles Me.FormClosing MyProcess.StandardInput.WriteLine("EXIT") 'send an EXIT command to the Command Prompt MyProcess.StandardInput.Flush() MyProcess.Close() End Sub Private Sub MyProcess_ErrorDataReceived(ByVal sender As Object, ByVal e As System.Diagnostics.DataReceivedEventArgs) Handles MyProcess.ErrorDataReceived AppendOutputText(vbCrLf & "Error: " & e.Data) End Sub Private Sub MyProcess_OutputDataReceived(ByVal sender As Object, ByVal e As System.Diagnostics.DataReceivedEventArgs) Handles MyProcess.OutputDataReceived AppendOutputText(vbCrLf & e.Data) End Sub Private Sub ExecuteButton_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ListBucketsButton.Click If PWTextBox.Text = "" Then MsgBox("Enter your password first") Else OutputTextBox.Text = "" MyProcess.StandardInput.WriteLine(InputTextBox.Text) MyProcess.StandardInput.WriteLine(PWTextBox.Text) MyProcess.StandardInput.Flush() End If End Sub Private Sub AppendOutputText(ByVal text As String) If OutputTextBox.InvokeRequired Then Dim myDelegate As New AppendOutputTextDelegate(AddressOf AppendOutputText) Me.Invoke(myDelegate, text) Else OutputTextBox.AppendText(text) End If End Sub Private Sub ImportKeysToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles ImportKeysToolStripMenuItem.Click Shell("storj import-keys", vbNormalFocus) End Sub Private Sub Button1_Click(sender As Object, e As EventArgs) Me.Close() End Sub Private Sub Button1_Click_1(sender As Object, e As EventArgs) Handles Button1.Click If BucketIDTextBox.Text = "" Then MsgBox("you must enter Bucket ID first") ElseIf UL2STextBox.Text = "" Then MsgBox("Use the browse button to find a file first. ...It's like you're not even trying :(") Else Shell("storj upload-file " & BucketIDTextBox.Text & " """ & UL2STextBox.Text & """", vbNormalFocus) End If UL2STextBox.Text = "" End Sub Private Sub Button2_Click(sender As Object, e As EventArgs) Handles ListFilesButton.Click If BucketIDTextBox.Text = "" Then MsgBox("you must enter Bucket ID first") Else OutputTextBox.Text = "" MyProcess.StandardInput.WriteLine(ListFIlesTextBox.Text & BucketIDTextBox.Text) MyProcess.StandardInput.WriteLine(PWTextBox.Text) MyProcess.StandardInput.Flush() End If End Sub Private Sub Button3_Click(sender As Object, e As EventArgs) Handles Button3.Click If BucketIDTextBox.Text = "" Then MsgBox("you must enter Bucket ID first") ElseIf FileIDTextBox.Text = "" Then MsgBox("Enter File ID") ElseIf DL2STextBox.Text = "" Then MsgBox("For Christ's sake, where do you want to save it???") Else Shell("storj download-file " & BucketIDTextBox.Text & " " & FileIDTextBox.Text & " """ & DL2STextBox.Text & """", vbNormalFocus) End If DL2STextBox.Text = "" FileIDTextBox.Text = "" End Sub Private Sub Button4_Click(sender As Object, e As EventArgs) Handles Button4.Click If FileIDTextBox.Text = "" Then MsgBox("you must enter File ID first") Else Shell("storj remove-file " & BucketIDTextBox.Text & " " & FileIDTextBox.Text & "", vbNormalFocus) End If FileIDTextBox.Text = "" End Sub Private Sub BrowseButton_Click(sender As Object, e As EventArgs) Handles BrowseButton.Click If OpenFileDialog1.ShowDialog = Windows.Forms.DialogResult.OK Then UL2STextBox.Text = OpenFileDialog1.FileName End If End Sub Private Sub CreateNewBucketToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles CreateNewBucketToolStripMenuItem.Click Form2.Show() End Sub End Class
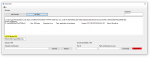