I am trying to complete a compound interest calculator with the ability to input the number of compounds per year. Here is what the form looks like:
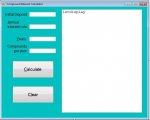
I will put the code below but here are my problems:
1) When entering a number in the compound box, all the program does is spit back my initial deposit.
2) When I enter the letter 'c' in the compound box, I get zeros for each year.
As always thank you for any help you can provide.
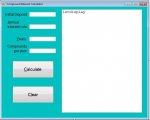
I will put the code below but here are my problems:
1) When entering a number in the compound box, all the program does is spit back my initial deposit.
2) When I enter the letter 'c' in the compound box, I get zeros for each year.
As always thank you for any help you can provide.
Public Class Form1 Dim dbldeposit As Double Dim intrate As Integer Dim intyears As Integer Dim strcompoundyears As String Dim dblyearendingbalance As Double Const STRFORMAT As String = "{0,-10}{1,-21:C}" Private Sub txtdeposit_KeyPress(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles txtdeposit.KeyPress If (e.KeyChar < "0" Or e.KeyChar > "9") And e.KeyChar <> Chr(8) And e.KeyChar <> Chr(36) And e.KeyChar <> "," Then e.KeyChar = Chr(0) MsgBox("Please enter a dollar amount.") 'restricts keys to 0-9, backspace, dollar sign, and the comma. End If End Sub Private Sub txtinterest_KeyPress(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles txtinterest.KeyPress If (e.KeyChar < "0" Or e.KeyChar > "9") And e.KeyChar <> Chr(8) And e.KeyChar <> "." And e.KeyChar <> "%" Then e.KeyChar = Chr(0) MsgBox("Please enter an interest rate.") 'restricts keys to 0-9, backspace, the period, and the percent sign. End If End Sub Private Sub txtyears_KeyPress(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles txtyears.KeyPress If (e.KeyChar < "0" Or e.KeyChar > "9") And e.KeyChar <> Chr(8) Then e.KeyChar = Chr(0) MsgBox("Please enter a number.") 'restricts keys to 0-9, letter c, and the backspace. End If End Sub Private Sub txtcompounds_KeyPress(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles txtcompounds.KeyPress If (e.KeyChar < "0" Or e.KeyChar > "9") And e.KeyChar <> Chr(99) And e.KeyChar <> Chr(8) Then e.KeyChar = Chr(0) MsgBox("Please enter a number or the letter c for continous compounds.") 'restricts keys to 0-9, letter c, and the backspace. End If End Sub Private Sub btncalculate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btncalculate.Click dbldeposit = txtdeposit.Text intrate = txtinterest.Text intyears = txtyears.Text strcompoundyears = txtcompounds.Text If intrate > 1 Then intrate = intrate / 100 End If lstdisplay.Items.Add(String.Format(STRFORMAT, "Year", "Year-ending balance")) For i As Integer = 1 To intyears If IsNumeric(strcompoundyears) Then dblyearendingbalance = dbldeposit * ((1 + intrate / strcompoundyears) ^ (strcompoundyears * i)) Else dblyearendingbalance = dbldeposit * (intrate / Math.Exp(intrate * intyears)) End If lstdisplay.Items.Add(String.Format(STRFORMAT, i, dblyearendingbalance)) Next End Sub Function IsGoodInput(ByVal input As Integer) As Boolean If input = "" Then Return False Else Return True End If End Function Private Sub btnclear_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnclear.Click txtdeposit.Clear() txtinterest.Clear() txtyears.Clear() txtcompounds.Clear() lstdisplay.Items.Clear() txtdeposit.Focus() End Sub End Class
Last edited by a moderator: