nK0de
Active member
This is not really a question. More like a clarification. I wanna know As to why this is happening.
This is an exercise from a tutorial which I did last year. I came across this today and thought of just trying it out. Its about using a DataSet and how you can make changes in it and update the database through it. Now the original exercise was done using Data controls such as SqlDataAdapter and generating DataSet through that. This is the form.
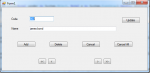
And this is the code for that.
But this time I thought of doing it through code.
Everything works fine but my doubt is with the updating part.
If I don't build a command using the SqlCommandBuilder, it throws the error Update requires a valid UpdateCommand when passed DataRow collection with modified rows. Note that I didn't have to do so when I was using Data controls.
Can anyone please explain why this is happening? I'm a little skeptical about using the SqlCommandBuilder bacause I've heard that although it works well with small queries, when it comes to complicated ones, its not very ideal. So is there any other way I can go about this?
I know its lengthy. Sorry about that. I need to clear up my mind on this. Thank you all.
This is an exercise from a tutorial which I did last year. I came across this today and thought of just trying it out. Its about using a DataSet and how you can make changes in it and update the database through it. Now the original exercise was done using Data controls such as SqlDataAdapter and generating DataSet through that. This is the form.
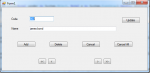
And this is the code for that.
Public Class Form1 Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load SqlDataAdapter1.Fill(DataSet11) End Sub Private Sub btnFirst_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnFirst.Click BindingContext(DataSet11, "master").Position = 0 End Sub Private Sub btnPrev_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnPrev.Click BindingContext(DataSet11, "master").Position -= 1 End Sub Private Sub btnNext_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnNext.Click BindingContext(DataSet11, "master").Position += 1 End Sub Private Sub btnLast_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnLast.Click BindingContext(DataSet11, "master").Position = BindingContext(DataSet11, "master").Count - 1 End Sub Private Sub btnAdd_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnAdd.Click BindingContext(DataSet11, "master").AddNew() End Sub Private Sub btnUpdate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnUpdate.Click Dim chDataset As DataSet BindingContext(DataSet11, "master").EndCurrentEdit() If DataSet11.HasChanges Then chDataset = DataSet11.GetChanges SqlDataAdapter1.Update(chDataset) End If DataSet11.AcceptChanges() End Sub Private Sub btnDelete_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnDelete.Click BindingContext(DataSet11, "master").RemoveAt(BindingContext(DataSet11, "master").Position) End Sub Private Sub btnCancel_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCancel.Click BindingContext(DataSet11, "master").CancelCurrentEdit() End Sub Private Sub btnCancelAll_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCancelAll.Click DataSet11.RejectChanges() End Sub End Class
But this time I thought of doing it through code.
Imports System.Data Imports System.Data.SqlClient Public Class Form4 Dim constr As String = "Data Source=NK0DE-PC;Initial Catalog=stockcontrol;User Id=sa;Password=abc123;" Dim ds As New DataSet() Dim adap As New SqlDataAdapter() Private Sub Form4_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load Dim sqlcon As New SqlConnection(constr) sqlcon.Open() adap.SelectCommand = New SqlCommand("SELECT * FROM Master", sqlcon) adap.Fill(ds, "mst") txtCode.DataBindings.Add("Text", ds, "mst.Code") txtName.DataBindings.Add("Text", ds, "mst.Name") End Sub Private Sub btnFirst_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnFirst.Click BindingContext(ds, "mst").Position = 0 End Sub Private Sub btnPrev_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnPrev.Click BindingContext(ds, "mst").Position -= 1 End Sub Private Sub btnNext_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnNext.Click BindingContext(ds, "mst").Position += 1 End Sub Private Sub btnLast_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnLast.Click BindingContext(ds, "mst").Position = BindingContext(ds, "mst").Count - 1 End Sub Private Sub btnAdd_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnAdd.Click BindingContext(ds, "mst").AddNew() End Sub Private Sub btnUpdate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnUpdate.Click 'updating the dataset with the new data Dim newData As DataSet BindingContext(ds, "mst").EndCurrentEdit() If ds.HasChanges Then newData = ds.GetChanges Dim combuild As New SqlCommandBuilder(adap) adap.Update(newData, "mst") End If ds.AcceptChanges() End Sub Private Sub btnDelete_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnDelete.Click BindingContext(ds, "mst").RemoveAt(BindingContext(ds, "mst").Position) End Sub Private Sub btnCancel_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCancel.Click BindingContext(ds, "mst").CancelCurrentEdit() End Sub Private Sub btnCancelAll_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCancelAll.Click ds.RejectChanges() End Sub End Class
Everything works fine but my doubt is with the updating part.
Private Sub btnUpdate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnUpdate.Click 'updating the dataset with the new data Dim newData As DataSet BindingContext(ds, "mst").EndCurrentEdit() If ds.HasChanges Then newData = ds.GetChanges 'Dim combuild As New SqlCommandBuilder(adap) adap.Update(newData, "mst") 'Update requires a valid UpdateCommand when passed DataRow collection with modified rows. End If ds.AcceptChanges() End Sub
If I don't build a command using the SqlCommandBuilder, it throws the error Update requires a valid UpdateCommand when passed DataRow collection with modified rows. Note that I didn't have to do so when I was using Data controls.
Can anyone please explain why this is happening? I'm a little skeptical about using the SqlCommandBuilder bacause I've heard that although it works well with small queries, when it comes to complicated ones, its not very ideal. So is there any other way I can go about this?
I know its lengthy. Sorry about that. I need to clear up my mind on this. Thank you all.