I've got a question that I hope someone can answer for me...At my place of work we have one person that creates programs for the other employees to use. Because of the nature of the work there are generally errors, and the process of reporting them is rather fuzzy.
What i want to do is create a few text boxes so that users can input data such as descriptions of errors, and for that data to be saved into its own new text file using one of the fields as a file name (saved into a specific directory). So ultimately there would be a folder with a list of text files containing errors to be dealt with. I'm doing this in visual Basic Express by the way.
I've made a quick mock up to illustrate what I'm trying to do...
So when the data is entered, the 'OK' button would save it to a .txt file.
Can anyone point me in the right direction with this?
What i want to do is create a few text boxes so that users can input data such as descriptions of errors, and for that data to be saved into its own new text file using one of the fields as a file name (saved into a specific directory). So ultimately there would be a folder with a list of text files containing errors to be dealt with. I'm doing this in visual Basic Express by the way.
I've made a quick mock up to illustrate what I'm trying to do...
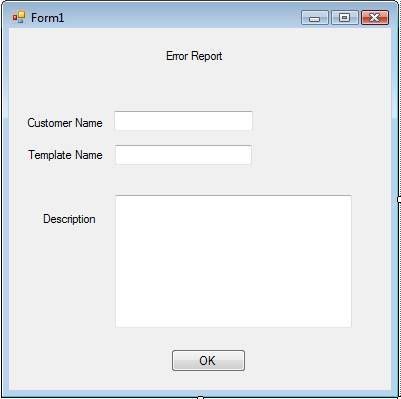
So when the data is entered, the 'OK' button would save it to a .txt file.
Can anyone point me in the right direction with this?
Last edited: