Im currently teaching myself to be familiar with this language
Right now im familiarizing some techniques and I am stuck at this dilemma.
Can anyone help me or suggest some tips? I am looking through the net but somehow I cant manage to find any tips so Im hoping that anyone here can help me...
As a practice, Im creating a simple program that can add and subtract two integer. But Im putting some user constraints.
My dilemmas are these, I want to have a text in the text box saying instructions to the user.
The other is I want to disable the two operating buttons which are ADD and SUBTRACT if there is no data input.
Like this, see how the text in the text boxes are not in regular color... So as the Add and Subtract buttons.
NOTE: I just edited this pic but this is how my app should be
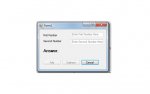
Then, when a data has been inserted, the text will be what the user had entered on the textbox and the
two operating buttons shall be enabled...
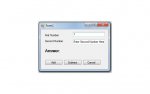
Any Tips would help... Thanks...
Right now im familiarizing some techniques and I am stuck at this dilemma.
Can anyone help me or suggest some tips? I am looking through the net but somehow I cant manage to find any tips so Im hoping that anyone here can help me...
As a practice, Im creating a simple program that can add and subtract two integer. But Im putting some user constraints.
My dilemmas are these, I want to have a text in the text box saying instructions to the user.
The other is I want to disable the two operating buttons which are ADD and SUBTRACT if there is no data input.
Like this, see how the text in the text boxes are not in regular color... So as the Add and Subtract buttons.
NOTE: I just edited this pic but this is how my app should be
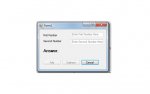
Then, when a data has been inserted, the text will be what the user had entered on the textbox and the
two operating buttons shall be enabled...
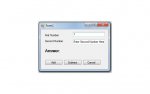
Any Tips would help... Thanks...