ikantspelwurdz
Well-known member
- Joined
- Dec 8, 2009
- Messages
- 49
- Programming Experience
- 1-3
I wrote an application that by design, when minimized, goes to the tray, and will only show up as a normal form when you doubleclick the icon in the tray.
To do this, I added a NotifyIcon, and added these functions:
This works fine, but I want the program to start minimized to the tray. So I added this line to the very end of Form1_Load:
When I run my program in debug mode within Visual Studio, this works fine.
When I run the exe directly, the form does not hide properly. It "minimizes" by resizing to a very small size, and looks like this, fixed in the lower-left corner of my screen:
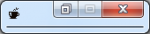
If I click the restore button, it restores and the restore button turns into a minimize button. If I click the minimize button, it minimizes to the tray correctly. But I want it to start that way. What is going wrong and how do I fix it?
To do this, I added a NotifyIcon, and added these functions:
VB.NET:
Private Sub Form1_Resize(sender As System.Object, e As System.EventArgs) Handles MyBase.Resize
If Me.WindowState = FormWindowState.Minimized Then
NotifyIcon1.Visible = True
ShowInTaskbar = False
Me.Hide()
End If
End Sub
Private Sub NotifyIcon1_DoubleClick(sender As System.Object, e As System.EventArgs) Handles NotifyIcon1.DoubleClick
Me.Show()
Me.WindowState = FormWindowState.Normal
NotifyIcon1.Visible = False
ShowInTaskbar = True
End Sub
This works fine, but I want the program to start minimized to the tray. So I added this line to the very end of Form1_Load:
VB.NET:
Me.WindowState = FormWindowState.Minimized
When I run my program in debug mode within Visual Studio, this works fine.
When I run the exe directly, the form does not hide properly. It "minimizes" by resizing to a very small size, and looks like this, fixed in the lower-left corner of my screen:
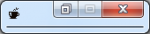
If I click the restore button, it restores and the restore button turns into a minimize button. If I click the minimize button, it minimizes to the tray correctly. But I want it to start that way. What is going wrong and how do I fix it?