Hello, I am creating my own money tracking program. I am in trouble at the part where save and load code of specific file starts.
I want to load and save my own created *.mmt files.
The files would be loaded and saved through Open / Save As window.
What code should I use to load these files?
What code should I use to save these files?
I'd like to save values of both textboxes into one file. Possible or not? If not - two files is not a problem though.
Also, if I can't use specific file extension (*.mmt), a general *.txt file won't ruin my app :tennis:
There is my code
=============================================================================================================================
Public Class Form1
Private Sub ImportToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles ImportToolStripMenuItem.Click
OpenFileDialog1.ShowDialog()
End Sub
Private Sub SaveToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles SaveToolStripMenuItem.Click
SaveFileDialog1.ShowDialog()
End Sub
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
ListBox1.Items.Add(TextBox1.Text & " got on " & TextBox3.Text & "; " & TextBox2.Text)
End Sub
Private Sub Label13_Click(sender As Object, e As EventArgs) Handles Label13.Click
End Sub
Private Sub Button2_Click(sender As Object, e As EventArgs) Handles Button2.Click
ListBox2.Items.Add(TextBox6.Text & " spent for " & TextBox5.Text & " on " & TextBox4.Text)
End Sub
Private Sub ExitToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles ExitToolStripMenuItem.Click
If MessageBox.Show("Have you saved your work?", "Wait!", MessageBoxButtons.YesNo, MessageBoxIcon.Question) = Windows.Forms.DialogResult.Yes Then
Me.Close()
Else
MessageBox.Show("Please save your work!", "Wait!", MessageBoxButtons.OK, MessageBoxIcon.Information)
SaveFileDialog1.ShowDialog()
End If
End Sub
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
End Sub
End Class
=============================================================================================================================
And some photos
The listbox menu. Probably only Exit function is working without problems.
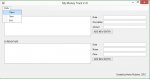
Open file window. Nothing happens even if I select *.mmt file (an empty file with *.mmt extension).
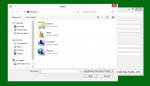
Added entries to both listboxes for a demonstration. Works perfectly.
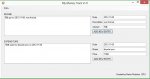
Save As file window. No file is saved even if I enter file name and click save.
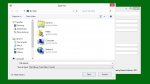
That's it.
Now, repeating again my questions:
What code should I use to load these files?
What code should I use to save these files?
Will be looking forward to answers!
I want to load and save my own created *.mmt files.
The files would be loaded and saved through Open / Save As window.
What code should I use to load these files?
What code should I use to save these files?
I'd like to save values of both textboxes into one file. Possible or not? If not - two files is not a problem though.
Also, if I can't use specific file extension (*.mmt), a general *.txt file won't ruin my app :tennis:
There is my code
=============================================================================================================================
Public Class Form1
Private Sub ImportToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles ImportToolStripMenuItem.Click
OpenFileDialog1.ShowDialog()
End Sub
Private Sub SaveToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles SaveToolStripMenuItem.Click
SaveFileDialog1.ShowDialog()
End Sub
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
ListBox1.Items.Add(TextBox1.Text & " got on " & TextBox3.Text & "; " & TextBox2.Text)
End Sub
Private Sub Label13_Click(sender As Object, e As EventArgs) Handles Label13.Click
End Sub
Private Sub Button2_Click(sender As Object, e As EventArgs) Handles Button2.Click
ListBox2.Items.Add(TextBox6.Text & " spent for " & TextBox5.Text & " on " & TextBox4.Text)
End Sub
Private Sub ExitToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles ExitToolStripMenuItem.Click
If MessageBox.Show("Have you saved your work?", "Wait!", MessageBoxButtons.YesNo, MessageBoxIcon.Question) = Windows.Forms.DialogResult.Yes Then
Me.Close()
Else
MessageBox.Show("Please save your work!", "Wait!", MessageBoxButtons.OK, MessageBoxIcon.Information)
SaveFileDialog1.ShowDialog()
End If
End Sub
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
End Sub
End Class
=============================================================================================================================
And some photos
The listbox menu. Probably only Exit function is working without problems.
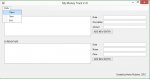
Open file window. Nothing happens even if I select *.mmt file (an empty file with *.mmt extension).
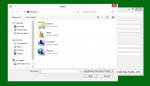
Added entries to both listboxes for a demonstration. Works perfectly.
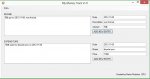
Save As file window. No file is saved even if I enter file name and click save.
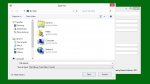
That's it.
Now, repeating again my questions:
What code should I use to load these files?
What code should I use to save these files?
Will be looking forward to answers!