topsykretts
Well-known member
- Joined
- Dec 22, 2011
- Messages
- 47
- Programming Experience
- Beginner
Hello,
I tried with code above but it removes only one duplicate by click.
As source I am using text file that has a words separated by ",". Content of text file: (Jacob,Jonah,Adam,Shawn,Aaron,Liam,Daniel,Ryan,Adam,Luke,Jacob)
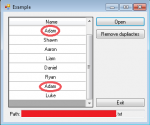
I tried with code above but it removes only one duplicate by click.
As source I am using text file that has a words separated by ",". Content of text file: (Jacob,Jonah,Adam,Shawn,Aaron,Liam,Daniel,Ryan,Adam,Luke,Jacob)
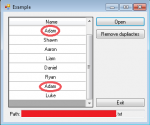
VB.NET:
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim Name As New DataGridViewTextBoxColumn
Name.Name = "Name"
Name.HeaderText = "Name"
Name.HeaderCell.Style.Alignment = DataGridViewContentAlignment.MiddleCenter
Name.DefaultCellStyle.Alignment = DataGridViewContentAlignment.MiddleCenter
Name.Width = 200
Name.SortMode = 0
DataGridView1.Columns.Add(Name)
End Sub
Private Sub btnOpen_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnOpen.Click
'Open TXT
Dim openFileDialog1 As New OpenFileDialog
openFileDialog1.InitialDirectory = System.Environment.GetFolderPath(Environment.SpecialFolder.Desktop)
openFileDialog1.Title = "Text Documents"
openFileDialog1.Filter = "Text Documents (*.txt)|*.txt"
openFileDialog1.FilterIndex = 1
openFileDialog1.RestoreDirectory = True
If openFileDialog1.ShowDialog() = DialogResult.OK Then
'Clear DGW
DataGridView1.Rows.Clear()
Dim stFilePathAndName As String = openFileDialog1.FileName
TextBox1.Text = stFilePathAndName
Dim sr As IO.StreamReader = New IO.StreamReader(stFilePathAndName)
Dim line As String
Do While Not sr.EndOfStream
line = sr.ReadLine()
Dim aryTextFile() As String
aryTextFile = line.Split(",")
For i = 0 To UBound(aryTextFile)
DataGridView1.Rows.Add(aryTextFile(i))
Next i
' Sort first column, ascending:
DataGridView1.Sort(DataGridView1.Columns(0), System.ComponentModel.ListSortDirection.Ascending)
If line = Nothing Then Continue Do ' This line skips blanks
Loop
sr.Close()
Else
openFileDialog1.Dispose()
End If
End Sub
Private Sub btnExit_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnExit.Click
Me.Close()
End Sub
Private Sub btnRemoveDuplicates_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnRemoveDuplicates.Click
For intI As Integer = 0 To DataGridView1.Rows.Count - 1
For intJ As Integer = intI + 1 To DataGridView1.Rows.Count - 1
If DataGridView1.Rows(intI).Cells(0).Value = DataGridView1.Rows(intJ).Cells(0).Value Then
DataGridView1.Rows.RemoveAt(intJ)
Exit Sub
End If
Next intJ
Next intI
End Sub