/*
SQL 2008
Visual Studio 2013 - Report Viewer
My vb skill level: Poor but I'm excited to learn
Profession: DBA
- Can I set up the sql connection in VB? - YES
- Can I set up the NAME, Data Source, and Table Adapter in VB? - YES
ERROR: Argument Not Specified For Parameter
*/
Please guys, I know how uncool it is to ask for a extra "hand holding", but I'm really new at this and realize I'm over my head so I would be very greatfull for your extra patience. Very Sincerely....
1. Here is my Form
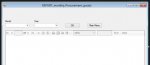
2. Here is my SELECT statement
SELECT * FROM dbo.procurement_goods WHERE MONTH(date) = @month AND YEAR(date) = @year
3. This is My Table Adapter
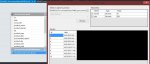
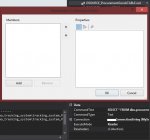
4. My Code in Visual Studio
I'm posting my new code here and my question to you is: what should my code look like to resolve the missing parameter?
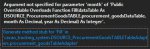
SQL 2008
Visual Studio 2013 - Report Viewer
My vb skill level: Poor but I'm excited to learn

Profession: DBA
- Can I set up the sql connection in VB? - YES
- Can I set up the NAME, Data Source, and Table Adapter in VB? - YES
ERROR: Argument Not Specified For Parameter
*/
Please guys, I know how uncool it is to ask for a extra "hand holding", but I'm really new at this and realize I'm over my head so I would be very greatfull for your extra patience. Very Sincerely....
1. Here is my Form
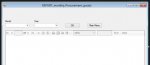
2. Here is my SELECT statement
SELECT * FROM dbo.procurement_goods WHERE MONTH(date) = @month AND YEAR(date) = @year
3. This is My Table Adapter
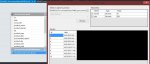
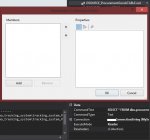
4. My Code in Visual Studio
I'm posting my new code here and my question to you is: what should my code look like to resolve the missing parameter?
Imports System Imports System.Data Imports System.Data.SqlClient Imports Microsoft.Reporting.WinForms Public Class REPORT_monthly_Procurement_goods Dim conn As SqlConnection Dim comm As SqlCommand Private Sub openconnection() conn = New SqlConnection conn.ConnectionString = "server=myserver;database=mydb;Integrated Security=SSPI;" conn.Open() End Sub Private Sub ComboBox_MONTH_SelectedIndexChanged(sender As Object, e As EventArgs) Handles ComboBox_MONTH.SelectedIndexChanged 'Convert Month Name to INT Dim var_month As String If ComboBox_MONTH.SelectedItem.ToString = "January" Then var_month = "1" If ComboBox_MONTH.SelectedItem.ToString = "February" Then var_month = "2" If ComboBox_MONTH.SelectedItem.ToString = "March" Then var_month = "3" If ComboBox_MONTH.SelectedItem.ToString = "April" Then var_month = "4" If ComboBox_MONTH.SelectedItem.ToString = "May" Then var_month = "5" If ComboBox_MONTH.SelectedItem.ToString = "June" Then var_month = "6" If ComboBox_MONTH.SelectedItem.ToString = "July" Then var_month = "7" If ComboBox_MONTH.SelectedItem.ToString = "August" Then var_month = "8" If ComboBox_MONTH.SelectedItem.ToString = "September" Then var_month = "9" If ComboBox_MONTH.SelectedItem.ToString = "October" Then var_month = "10" If ComboBox_MONTH.SelectedItem.ToString = "November" Then var_month = "11" If ComboBox_MONTH.SelectedItem.ToString = "December" Then var_month = "12" End Sub Private Sub ComboBox_YEAR_SelectedIndexChanged(sender As Object, e As EventArgs) Handles ComboBox_YEAR.SelectedIndexChanged End Sub Private Sub go_button_Click(sender As Object, e As EventArgs) Handles go_button.Click comm.Parameters.AddWithValue("@month", CInt(ComboBox_MONTH.Text)) comm.Parameters.AddWithValue("@year", CInt(ComboBox_YEAR.Text)) End Sub Private Sub REPORT_monthly_Procurement_goods_Load(sender As Object, e As EventArgs) Handles MyBase.Load 'Errors here: Me.procurement_goodsTableAdapter.Fill(Me.DSOURCE_ProcurementGoodsTABLE.procurement_goods) Me.ReportViewer1.RefreshReport() End Sub End Class
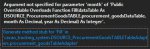