Hello all!
I'm new to VB.NET and have been toying around with creating functions, subs, classes, etc. One test program I've written tests the differences in processing times via two methods: variable access vs. function invocation.
What I mean by this is I have one module and one function. In the module I've declared a String object that is set by calling my function 'ReturnString()'. I also have two DateTime objects for recording elapsed seconds.
In one instance, I call 'Console.Writeline(MyString)' and report the time it took for that line to process. Then I called 'Console.WriteLine(ReturnString())' and reported the time it took to process that line.
My impression is that the first 'Console.WriteLine(MyString)' invocation would take up a much shorter time frame to execute than the 'Console.WriteLine(ReturnString())' call, however when running my program I found that to be false.
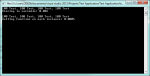
So, my question is such: How is it that TWO function invocations take less time than only one?
I'm new to VB.NET and have been toying around with creating functions, subs, classes, etc. One test program I've written tests the differences in processing times via two methods: variable access vs. function invocation.
What I mean by this is I have one module and one function. In the module I've declared a String object that is set by calling my function 'ReturnString()'. I also have two DateTime objects for recording elapsed seconds.
In one instance, I call 'Console.Writeline(MyString)' and report the time it took for that line to process. Then I called 'Console.WriteLine(ReturnString())' and reported the time it took to process that line.
My impression is that the first 'Console.WriteLine(MyString)' invocation would take up a much shorter time frame to execute than the 'Console.WriteLine(ReturnString())' call, however when running my program I found that to be false.
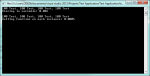
So, my question is such: How is it that TWO function invocations take less time than only one?
VB.NET:
Module Module1
Sub Main()
Dim TestInteger As Integer = 100
Dim TestString As String = ReturnString(TestInteger)
Dim StartTime As DateTime = New DateTime()
Dim EndTime As DateTime = New DateTime()
StartTime = DateTime.Now
Console.WriteLine(TestString)
EndTime = DateTime.Now
Console.WriteLine("Storing in variable: {0}", EndTime.Subtract(StartTime).TotalSeconds)
StartTime = DateTime.Now
Console.WriteLine(ReturnString(TestInteger))
EndTime = DateTime.Now
Console.WriteLine("Calling function on each instance: {0}", EndTime.Subtract(StartTime).TotalSeconds)
Console.Read()
End Sub
Function ReturnString(ByVal Int As Integer)
Dim MyString As String = Int.ToString
MyString = MyString & " Test"
Return MyString
End Function
End Module