paulthepaddy
Well-known member
Hi guys, im hoping some one will be able to help me with this problem.
Here is my tables
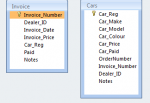
I dont really want to use a many to many but if i have to, just say
As far as i know and have read, MS Access allows for multiple values in a single field, but i cant get it to work.
an invoice will have multiple cars on it, and for most parts each car shall only be on 1 invoice, but often enough it will appear on 2 so really this is a many to many
The relationship works when an invoice only have 1 reg on it, but it wont show ANY when it has 2 and the same goes for cars when it has 2 invoice numbers in its invoice field.
if i have to go the many to many relationship route then how do i use that in VB.NET
It Will be as follows
Selecting a car Should pull the details of the invoice its on, so this is sometimes 2 or more BUT selecting an invoice should pull all cars even if they appear on mroe than 1 invoice
Thanks For any help in advance
Here is my tables
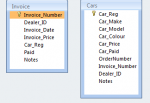
I dont really want to use a many to many but if i have to, just say
As far as i know and have read, MS Access allows for multiple values in a single field, but i cant get it to work.
an invoice will have multiple cars on it, and for most parts each car shall only be on 1 invoice, but often enough it will appear on 2 so really this is a many to many
The relationship works when an invoice only have 1 reg on it, but it wont show ANY when it has 2 and the same goes for cars when it has 2 invoice numbers in its invoice field.
if i have to go the many to many relationship route then how do i use that in VB.NET
It Will be as follows
Selecting a car Should pull the details of the invoice its on, so this is sometimes 2 or more BUT selecting an invoice should pull all cars even if they appear on mroe than 1 invoice
Thanks For any help in advance