Coolbreeze78
Member
- Joined
- Mar 15, 2013
- Messages
- 13
- Programming Experience
- 1-3
I am fairly new to Visual Basic.Net and am working on a network simulator program to simulate packets being sent across a network. I do have experience in Java however but I couldn't get the code to do what I wanted so I switched to an easier language for GUIs.
My problem is that I have an array of circles that act as place holders on the screen, that I light up green when the packet is at that current location once the simulation is ran. I have a for loop that goes through the array and obtains the x,y coordinates of the current circle in the array.
What I am trying to do is, once the first circle is drawn green, wait for the user to press the space bar to continue execution and advance the loop to draw the next green circle. I have tried several ways of doing this by browsing the internet but I can't get it to work. I will past the code I have as well as the screenshot of the GUI to provide an idea of what I am trying to do.
Any help would be greatly appreciated as I am currently stuck and don't know what else to do besides work through my Visual Basic 2010 In 24 Hours book to try and learn as much as I can in hopes that I can apply that knowledge at a future time.
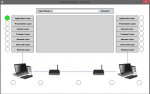
My problem is that I have an array of circles that act as place holders on the screen, that I light up green when the packet is at that current location once the simulation is ran. I have a for loop that goes through the array and obtains the x,y coordinates of the current circle in the array.
What I am trying to do is, once the first circle is drawn green, wait for the user to press the space bar to continue execution and advance the loop to draw the next green circle. I have tried several ways of doing this by browsing the internet but I can't get it to work. I will past the code I have as well as the screenshot of the GUI to provide an idea of what I am trying to do.
Any help would be greatly appreciated as I am currently stuck and don't know what else to do besides work through my Visual Basic 2010 In 24 Hours book to try and learn as much as I can in hopes that I can apply that knowledge at a future time.
VB.NET:
Public Class NetworkSimulator
Private intCircles(19, 2) As Integer
Private flag As Boolean
' Create graphics object
Dim g As Graphics
Private Sub NetworkSimulator_Paint(sender As Object, e As System.Windows.Forms.PaintEventArgs) Handles Me.Paint
' Create graphics
g = Me.CreateGraphics
' Sizes
Dim boxHeight, boxWidth, circleHeight, circleWidth, lboxX, rboxX, boxY, lcircleX, rcircleX, circleY As Integer
boxHeight = 35
boxWidth = 150
circleHeight = 30
circleWidth = 30
' Positions
lboxX = 75
rboxX = 782
boxY = 75
lcircleX = 30
rcircleX = 941
circleY = 75
' Populate the cirlces array
intCircles(0, 0) = 30
intCircles(0, 1) = 75
intCircles(1, 0) = 30
intCircles(1, 1) = 110
intCircles(2, 0) = 30
intCircles(2, 1) = 145
intCircles(3, 0) = 30
intCircles(3, 1) = 180
intCircles(4, 0) = 30
intCircles(4, 1) = 215
intCircles(5, 0) = 30
intCircles(5, 1) = 250
intCircles(6, 0) = 30
intCircles(6, 1) = 285
' Middle set
intCircles(7, 0) = 70
intCircles(7, 1) = 510
intCircles(8, 0) = 255
intCircles(8, 1) = 510
intCircles(9, 0) = 512
intCircles(9, 1) = 510
intCircles(10, 0) = 739
intCircles(10, 1) = 510
intCircles(11, 0) = 924
intCircles(11, 1) = 510
' Right set
intCircles(12, 0) = 941
intCircles(12, 1) = 75
intCircles(13, 0) = 941
intCircles(13, 1) = 110
intCircles(14, 0) = 941
intCircles(14, 1) = 145
intCircles(15, 0) = 941
intCircles(15, 1) = 180
intCircles(16, 0) = 941
intCircles(16, 1) = 215
intCircles(17, 0) = 941
intCircles(17, 1) = 250
intCircles(18, 0) = 941
intCircles(18, 1) = 285
' Draw circles
Dim cX, cY As Integer
Dim circlePen As New Pen(Color.Black, 2)
For i As Integer = 0 To 18
For j As Integer = 0 To 0
cX = intCircles(i, j)
j += 1
cY = intCircles(i, j)
g.DrawEllipse(Pens.Black, cX, cY, circleWidth, circleHeight)
Next
circleY += circleHeight + 5
Next
'Draw(Boxes)
For index As Integer = 0 To 6
g.DrawRectangle(Pens.Black, lboxX, boxY, boxWidth, boxHeight)
g.DrawRectangle(Pens.Black, rboxX, boxY, boxWidth, boxHeight)
g.FillRectangle(Brushes.LightGray, lboxX + 1, boxY + 1, boxWidth - 1, boxHeight - 1)
g.FillRectangle(Brushes.LightGray, rboxX + 1, boxY + 1, boxWidth - 1, boxHeight - 1)
boxY += boxHeight
Next
' Set Font
Dim objFont As Font
objFont = New System.Drawing.Font("Arial Black", 8)
' Fill in Layer Text
g.DrawString("Application Layer", objFont, System.Drawing.Brushes.Black, 97, 86)
g.DrawString("Application Layer", objFont, System.Drawing.Brushes.Black, 804, 86)
g.DrawString("Presentation Layer", objFont, System.Drawing.Brushes.Black, 95, 122)
g.DrawString("Presentation Layer", objFont, System.Drawing.Brushes.Black, 802, 122)
g.DrawString("Session Layer", objFont, System.Drawing.Brushes.Black, 107, 157)
g.DrawString("Session Layer", objFont, System.Drawing.Brushes.Black, 814, 157)
g.DrawString("Transport Layer", objFont, System.Drawing.Brushes.Black, 103, 192)
g.DrawString("Transport Layer", objFont, System.Drawing.Brushes.Black, 810, 192)
g.DrawString("Network Layer", objFont, System.Drawing.Brushes.Black, 107, 227)
g.DrawString("Network Layer", objFont, System.Drawing.Brushes.Black, 814, 227)
g.DrawString("Data Link Layer", objFont, System.Drawing.Brushes.Black, 104, 262)
g.DrawString("Data Link Layer", objFont, System.Drawing.Brushes.Black, 811, 262)
g.DrawString("Physical Layer", objFont, System.Drawing.Brushes.Black, 108, 297)
g.DrawString("Physical Layer", objFont, System.Drawing.Brushes.Black, 815, 297)
' Draw display info box and input placeholder
g.DrawRectangle(Pens.Black, 242, 75, 523, 245)
g.DrawRectangle(Pens.Black, 242, 25, 523, boxHeight)
g.FillRectangle(Brushes.LightGray, 243, 76, 522, 244)
g.FillRectangle(Brushes.LightGray, 243, 26, 522, boxHeight - 1)
' Draw network cables
g.DrawLine(Pens.Blue, 150, 320, 150, 397)
g.DrawLine(Pens.Blue, 857, 320, 857, 397)
g.DrawLine(Pens.Blue, 207, 450, 328, 450)
g.DrawLine(Pens.Blue, 400, 450, 628, 450)
g.DrawLine(Pens.Blue, 700, 450, 810, 450)
End Sub
Private Sub btnInput_Click(sender As System.Object, e As System.EventArgs) Handles btnInput.Click
' Sizes
Dim cX, cY, circleHeight, circleWidth As Integer
circleHeight = 30
circleWidth = 30
' Loop through the array when the user presses space and display the appropriate content
For i As Integer = 0 To 18
For j As Integer = 0 To 0
cX = intCircles(i, j)
j += 1
cY = intCircles(i, j)
' Display a green circle over a black one
g.FillEllipse(Brushes.Black, cX, cY, circleWidth, circleHeight)
g.FillEllipse(Brushes.Lime, cX + 1, cY + 1, circleWidth - 2, circleHeight - 2)
Next
' Display Data for the current layer
' Wait for user to press Space Bar
flag = False
Call Wait()
Next
End Sub
Private Sub NetworkSimulator_KeyPress(sender As Object, e As System.Windows.Forms.KeyPressEventArgs) Handles Me.KeyPress
If e.KeyChar = Chr(32) Then
flag = True
End If
End Sub
Public Sub Wait()
Do While flag = False
Application.DoEvents()
Loop
End Sub
End Class
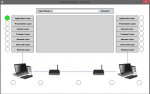