Jommaria03
New member
- Joined
- Dec 8, 2014
- Messages
- 1
- Programming Experience
- Beginner
I have here a code and could someone fix it?
I have here the code of the delete button.
How to delete the selected rows in datagridview and also be deleted in Ms access. The code here only deletes the record one by one starting from the first row not when selecting a row.
I don't know what code to add. please help...
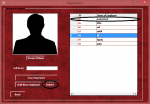
I have here the code of the delete button.
How to delete the selected rows in datagridview and also be deleted in Ms access. The code here only deletes the record one by one starting from the first row not when selecting a row.
I don't know what code to add. please help...
Private Sub DeleteData() Dim conn As New OleDbConnection Dim sConnString As String Dim cmd As New OleDbCommand Dim da As New OleDbDataAdapter Dim dt As New DataTable Try If Microsoft.VisualBasic.Right(Application.StartupPat h, 1) = "\" Then sConnString = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & Application.StartupPath & "SampleDB.accdb;Persist Security Info=False;" Else sConnString = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & Application.StartupPath & "\SampleDB.accdb;Persist Security Info=False;" End If conn = New OleDbConnection(sConnString) conn.Open() cmd.Connection = conn cmd.CommandType = CommandType.Text cmd.CommandText = "select id, image_description as description from tbl_image" da.SelectCommand = cmd da.Fill(dt) Me.DataGridView1.DataSource = dt dt.Rows(0).BeginEdit() dt.Rows(0).Delete() dt.Rows(0).EndEdit() Dim cb As New OleDbCommandBuilder(da) da.Update(dt) Me.DataGridView1.DataSource = dt.DefaultView Catch ex As Exception MsgBox(ErrorToString) Finally conn.Close() End Try End Sub
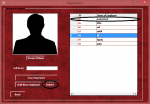