Coolbreeze78
Member
- Joined
- Mar 15, 2013
- Messages
- 13
- Programming Experience
- 1-3
I am once again stuck in my Network Simulator project that I have been working on and am in need of help. My problem this time is that I have two graphs that I am manually drawing on a picturebox, yes I know I could use images, but it is what it is. And yeah, I am not drawing on the Paint method of the picturebox because I have multiple method calls that draw different things that I use and I couldn't just move them all into the Paint method. So I have the graph drawn as shown below. But now I need the graph to scroll to the left either automatically or manually with scroll bars.
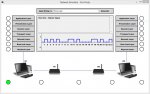
The problem I am having is that when I add a panel and put the picturebox into it, the scroll bars show up but when you scroll the image disappears. I also tried to use a while loop to animate the drawing on the picturebox and move it a few pixels and redraw the image but I get a lot of flickering then. Most likely because I am not in the Paint method. I actually tried to put the code into the Paint method, but I am using a reference to an array to draw aspects of the GUI, and I can't pass the reference to the Paint methods.
Anyway, I am pasting the code below so maybe someone can give me a hint at what I need to do to get the entire graph showing correctly within the area of the GUI.
Thanks guys!
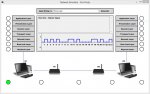
The problem I am having is that when I add a panel and put the picturebox into it, the scroll bars show up but when you scroll the image disappears. I also tried to use a while loop to animate the drawing on the picturebox and move it a few pixels and redraw the image but I get a lot of flickering then. Most likely because I am not in the Paint method. I actually tried to put the code into the Paint method, but I am using a reference to an array to draw aspects of the GUI, and I can't pass the reference to the Paint methods.
Anyway, I am pasting the code below so maybe someone can give me a hint at what I need to do to get the entire graph showing correctly within the area of the GUI.
Thanks guys!
VB.NET:
Public Class NetworkSimulator
' Declare globals
Private intCounter As Integer = 0 ' Determines the current location in the array of the current packet
Private strInput As String ' Stores the string that the user enters
Private strBinary As String ' Stores the binary representation of the input string
Private strDecimal As String ' Stores the decimal representation of the input string
Private enterFlag As Boolean = False ' Determines if this is the first or subsequent press of the enter button
Private binArray() As Integer ' Stores the values of the binary string in an array
Private preamble As String = "01010101010101010101010101010101010101010101010101010101" ' Stores the preamble for the ethernet frame
Private sfd As String = "10101011" ' Stores the start frame delimiter
Private destinationAddress As String ' Stores the destination address
Private sourceAddress As String ' Stores the source address
Private type As String = "0101110011001100" ' Stores the type
Private crc As String ' Stores the CRC for error checking
'=========================================================================================================================================
Private Sub NetworkSimulator_KeyDown(sender As Object, e As System.Windows.Forms.KeyEventArgs) Handles Me.KeyDown
If (e.KeyCode = Keys.Enter) And (enterFlag = True) Then
' Advance the packet
intCounter = AdvancePos(intCounter)
ElseIf (e.KeyCode = Keys.Enter) And (enterFlag = False) Then
btnInput_Click(sender, New System.EventArgs())
End If
End Sub
'=========================================================================================================================================
Private Sub NetworkSimulator_Paint(sender As Object, e As System.Windows.Forms.PaintEventArgs) Handles Me.Paint
' Create graphics
Dim g As Graphics
g = Me.CreateGraphics
' Draw network cables
g.DrawLine(Pens.Blue, 150, 320, 150, 397)
g.DrawLine(Pens.Blue, 857, 320, 857, 397)
g.DrawLine(Pens.Blue, 207, 450, 328, 450)
g.DrawLine(Pens.Blue, 400, 450, 628, 450)
g.DrawLine(Pens.Blue, 700, 450, 810, 450)
'Clean up the memory
g.Dispose()
End Sub
'=========================================================================================================================================
Private Sub btnInput_Click(sender As System.Object, e As System.EventArgs) Handles btnInput.Click
' Enable the flag to show the first enter was hit
enterFlag = True
' Remove focus from textbox
lblInput.Focus()
' Get the string
strInput = txtInput.Text
' Advance the packet
intCounter = AdvancePos(intCounter)
End Sub
'=========================================================================================================================================
Private Function AdvancePos(ByVal pos As Integer) As Integer
If strInput <> String.Empty Then
' Is this a new packet, if so start at position 0
If pos = 0 Then
' Convert input string to binary
strBinary = convertToBinary(strInput)
' Make a temp array to store the newly formated values
Dim tempArray(strBinary.Length - 1) As Integer
' Place binary numbers into an array
For index As Integer = 0 To strBinary.Length - 1
tempArray(index) = If(strBinary.Chars(index) = "0", 0, 1)
Next
' Pass the array reference to the real array
binArray = tempArray
' Swap the image
picCircle0.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Application Layer" & vbCrLf
' Advance the position of current
pos += 1
' If this is a current packet, advance the position
Else
' Check the new position to make sure it doesn't go past position 18
If pos < 19 Then
If pos = 1 Then
' Swap the previous circle
picCircle0.Image = My.Resources.CircleClear
' Swap the current circle
picCircle1.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Presentation Layer" & vbCrLf
ElseIf pos = 2 Then
' Swap the previous circle
picCircle1.Image = My.Resources.CircleClear
' Swap the current circle
picCircle2.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Session Layer" & vbCrLf
ElseIf pos = 3 Then
' Swap the previous circle
picCircle2.Image = My.Resources.CircleClear
' Swap the current circle
picCircle3.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Transport Layer" & vbCrLf
ElseIf pos = 4 Then
' Swap the previous circle
picCircle3.Image = My.Resources.CircleClear
' Swap the current circle
picCircle4.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Network Layer" & vbCrLf
ElseIf pos = 5 Then
' Swap the previous circle
picCircle4.Image = My.Resources.CircleClear
' Swap the current circle
picCircle5.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Data Link Layer" & vbCrLf
txtInfo.Text += "Preamble: " & preamble & vbCrLf
txtInfo.Text += "SFD: " & sfd & vbCrLf
txtInfo.Text += "Destination Address: " & destinationAddress & vbCrLf
txtInfo.Text += "Source Address: " & sourceAddress & vbCrLf
txtInfo.Text += "Type: " & type & vbCrLf
txtInfo.Text += "Data / Padding: " & "Insert Data Here" & vbCrLf
txtInfo.Text += "CRC: " & crc & vbCrLf
' Clear the previous graph
clearGraph()
' Display the ethernet frame picture
DrawEthernetFrame()
ElseIf pos = 6 Then
' Swap the previous circle
picCircle5.Image = My.Resources.CircleClear
' Swap the current circle
picCircle6.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Physical Layer" & vbCrLf
' Display the string
txtInfo.Text += "String: " & strInput & vbCrLf
' Display the conversion
txtInfo.Text += "Binary: " & strBinary
' Clear the previous graph
clearGraph()
ElseIf pos = 7 Then
' Swap the previous circle
picCircle6.Image = My.Resources.CircleClear
' Swap the current circle
picCircle7.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Host One - Digital Signal" & vbCrLf
' Clear the previous graph
clearGraph()
' Draw the digital graph
DrawDigitalGraph(binArray)
ElseIf pos = 8 Then
' Swap the previous circle
picCircle7.Image = My.Resources.CircleClear
' Swap the current circle
picCircle8.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Router One - Analog Signal" & vbCrLf
' Clear the previous graph
clearGraph()
' Draw the analog graph
DrawAnalogGraph(binArray)
ElseIf pos = 9 Then
' Swap the previous circle
picCircle8.Image = My.Resources.CircleClear
' Swap the current circle
picCircle9.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Midpoint - Analog Signal" & vbCrLf
' Clear the previous graph
clearGraph()
' Draw the analog graph
DrawAnalogGraph(binArray)
ElseIf pos = 10 Then
' Swap the previous circle
picCircle9.Image = My.Resources.CircleClear
' Swap the current circle
picCircle10.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Router Two - Analog Signal" & vbCrLf
' Clear the previous graph
clearGraph()
' Draw the analog graph
DrawAnalogGraph(binArray)
ElseIf pos = 11 Then
' Swap the previous circle
picCircle10.Image = My.Resources.CircleClear
' Swap the current circle
picCircle11.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Host Two - Digital Signal" & vbCrLf
' Clear the previous graph
clearGraph()
' Draw the digital graph
DrawDigitalGraph(binArray)
ElseIf pos = 12 Then
' Swap the previous circle
picCircle11.Image = My.Resources.CircleClear
' Swap the current circle
picCircle12.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Physical Layer" & vbCrLf
' Display the string
txtInfo.Text += "Binary: " & strBinary & vbCrLf
' Convert input string to binary and display it
strDecimal = convertToString(strBinary)
' Display the conversion
txtInfo.Text += "String: " & strDecimal
' Clear the previous graph
clearGraph()
ElseIf pos = 13 Then
' Swap the previous circle
picCircle12.Image = My.Resources.CircleClear
' Swap the current circle
picCircle13.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Data Link Layer" & vbCrLf
' Clear the previous graph
clearGraph()
' Display the ethernet frame picture
DrawEthernetFrame()
ElseIf pos = 14 Then
' Swap the previous circle
picCircle13.Image = My.Resources.CircleClear
' Swap the current circle
picCircle14.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Network Layer" & vbCrLf
' Clear the previous graph
clearGraph()
ElseIf pos = 15 Then
' Swap the previous circle
picCircle14.Image = My.Resources.CircleClear
' Swap the current circle
picCircle15.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Transport Layer" & vbCrLf
ElseIf pos = 16 Then
' Swap the previous circle
picCircle15.Image = My.Resources.CircleClear
' Swap the current circle
picCircle16.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Session Layer" & vbCrLf
ElseIf pos = 17 Then
' Swap the previous circle
picCircle16.Image = My.Resources.CircleClear
' Swap the current circle
picCircle17.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Presentation Layer" & vbCrLf
ElseIf pos = 18 Then
' Swap the previous circle
picCircle17.Image = My.Resources.CircleClear
' Swap the current circle
picCircle18.Image = My.Resources.CircleGreen
' Clear the previous info
txtInfo.Text = ""
' Display the info for this layer
txtInfo.Text = "Application Layer" & vbCrLf
End If
Else
' Reset the current position to 0
pos = 0
' Clear the last circle
picCircle18.Image = My.Resources.CircleClear
' Set input to null
txtInput.Text = ""
' Clear the textbox
txtInfo.Text = ""
' Clear the graph
clearGraph()
' Give focus to textbook
txtInput.Focus()
' Reset the flag for the first enter
enterFlag = False
' Exit loop and return
Return pos
End If
' Advance the position of current
pos += 1
End If
End If
' Return the new current position
Return pos
End Function
'=========================================================================================================================================
Private Function convertToBinary(ByVal str As String) As String
' Convert string to binary
Dim Result As String = ""
For Each C As Char In str
Dim s As String = System.Convert.ToString(AscW(C), 2).PadLeft(8, "0")
Result &= s
Next
Return Result
End Function
'=========================================================================================================================================
Private Function convertToString(ByVal str As String) As String
' Convert binary to string
Dim Result As String = ""
Dim I As Integer = 0
Dim J As Integer = 0
For Each C As Char In str.ToCharArray
J *= 2
If C = "1"c Then J += 1
I += 1
If I = 8 Then
I = 0
Result &= Chr(J)
J = 0
End If
Next
Return Result
End Function
'=========================================================================================================================================
Private Sub DrawEthernetFrame()
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create pens
Dim objThinPen = New Pen(Drawing.Color.Black, 2)
' Create Font
Dim objFont = New System.Drawing.Font("Arial Black", 7)
'510,115
'480,30
' Draw the background colors
g.FillRectangle(Brushes.Orange, 10, 30, 80, 40)
g.FillRectangle(Brushes.Lime, 90, 30, 65, 40)
g.FillRectangle(Brushes.Lime, 90, 30, 65, 40)
g.FillRectangle(Brushes.LightSkyBlue, 155, 30, 70, 40)
g.FillRectangle(Brushes.Yellow, 225, 30, 65, 40)
g.FillRectangle(Brushes.Red, 290, 30, 130, 40)
g.FillRectangle(Brushes.Black, 420, 30, 80, 40)
' Draw the ethernet frame lines
g.DrawRectangle(objThinPen, 10, 30, 490, 40)
g.DrawLine(objThinPen, 65, 30, 65, 70)
g.DrawLine(objThinPen, 90, 30, 90, 70)
g.DrawLine(objThinPen, 155, 30, 155, 70)
g.DrawLine(objThinPen, 225, 30, 225, 70)
g.DrawLine(objThinPen, 290, 30, 290, 70)
g.DrawLine(objThinPen, 420, 30, 420, 70)
' Draw labels over boxes
g.DrawString("Preamble", objFont, System.Drawing.Brushes.Black, 12, 43)
g.DrawString("S", objFont, System.Drawing.Brushes.Black, 72, 33)
g.DrawString("F", objFont, System.Drawing.Brushes.Black, 72, 43)
g.DrawString("D", objFont, System.Drawing.Brushes.Black, 72, 53)
g.DrawString("Destination", objFont, System.Drawing.Brushes.Black, 92, 37)
g.DrawString("Address", objFont, System.Drawing.Brushes.Black, 100, 50)
g.DrawString("Source", objFont, System.Drawing.Brushes.Black, 170, 37)
g.DrawString("Address", objFont, System.Drawing.Brushes.Black, 168, 50)
g.DrawString("Type", objFont, System.Drawing.Brushes.Black, 243, 43)
g.DrawString("Data / Payload", objFont, System.Drawing.Brushes.White, 317, 43)
g.DrawString("Frame Check", objFont, System.Drawing.Brushes.White, 425, 32)
g.DrawString("Sequence", objFont, System.Drawing.Brushes.White, 432, 43)
g.DrawString("(CRC)", objFont, System.Drawing.Brushes.White, 445, 54)
End Sub
'=========================================================================================================================================
Private Sub DrawDigitalGraph(ByRef refArray As Array)
' Set up positions for graph drawing
Dim xPos As Integer = 25
Dim yPos As Integer = 57
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create pens
Dim objThinPen = New Pen(Drawing.Color.Black)
' Create Font
Dim objFont = New System.Drawing.Font("Arial Black", 8)
' Get how many tics there will be
Dim numTics As Integer = refArray.Length
' Get total distance needed for graph
Dim totalWidth As Integer = (numTics * 20) + 50 '+ Me.Width
' Set ticPos
Dim ticPos As Integer = 1
' Create a temp xPos
Dim tempX As Integer = xPos
' Draw Digital Graph Baseline and Increments
g.DrawLine(objThinPen, tempX, 0, tempX, Me.Height)
g.DrawLine(objThinPen, tempX, yPos, totalWidth, yPos)
g.DrawString("1", objFont, System.Drawing.Brushes.Black, tempX - 12, 15)
g.DrawString("-1", objFont, System.Drawing.Brushes.Black, tempX - 17, 85)
' Draw tic marks
For index As Integer = 0 To numTics - 1
tempX += 20
g.DrawLine(objThinPen, tempX, yPos - 5, tempX, yPos + 5)
g.DrawString(ticPos, objFont, System.Drawing.Brushes.Black, tempX - 6, yPos + 10)
ticPos += 1
Next
' Reset tempX
tempX = xPos
' Draw the Digital Graph
For index As Integer = 0 To binArray.Length - 2
If binArray(index) = 0 Then
If binArray(index + 1) = 0 Then
' Draw Line 1 and increment the x position
Line1(tempX, yPos)
tempX += 20
Else
' Draw Line 2 and increment the x position
Line2(tempX, yPos)
tempX += 20
End If
Else
If binArray(index + 1) = 1 Then
' Draw Line 3 and increment the x position
Line3(tempX, yPos)
tempX += 20
Else
' Draw Line 4 and increment the x position
Line4(tempX, yPos)
tempX += 20
End If
End If
Next
' Fix the last line
If binArray(binArray.Length - 2) = 0 Then
If binArray(binArray.Length - 1) = 0 Then
' Draw Line 1
Line1(tempX, yPos)
Else
' Draw Line 4
Line4(tempX, yPos)
End If
Else
If binArray(binArray.Length - 1) = 1 Then
' Draw Line 4
Line4(tempX, yPos)
tempX += 20
Else
' Draw Line 1
Line1(tempX, yPos)
tempX += 20
End If
End If
' Clean up memory
objThinPen.Dispose()
objFont.Dispose()
g.Dispose()
End Sub
'=========================================================================================================================================
Private Sub DrawAnalogGraph(ByRef refArray As Array)
' Set up positions for graph drawing
Dim xPos As Integer = 25
Dim yPos As Integer = 57
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create pens
Dim objThinPen = New Pen(Drawing.Color.Black)
Dim objThickPen = New Pen(Drawing.Color.Blue, 2)
' Create Font
Dim objFont = New System.Drawing.Font("Arial Black", 8)
' Get how many tics there will be
Dim numTics As Integer = refArray.Length
' Get total distance needed for graph
Dim totalLength As Integer = (numTics * 40) + 50 + Me.Width
' Set ticPos
Dim ticPos As Integer = 1
' Draw Digital Graph Baseline and Increments
g.DrawLine(objThinPen, xPos, 0, xPos, Me.Height)
g.DrawLine(objThinPen, xPos, yPos, totalLength, yPos)
g.DrawString("1", objFont, System.Drawing.Brushes.Black, 13, 15)
g.DrawString("-1", objFont, System.Drawing.Brushes.Black, 8, 85)
' Draw tic marks
For index As Integer = 0 To numTics - 1
xPos += 40
g.DrawLine(objThinPen, xPos, yPos - 5, xPos, yPos + 5)
g.DrawString(ticPos, objFont, System.Drawing.Brushes.Black, xPos - 6, yPos + 37)
ticPos += 1
Next
' Reset xpos
xPos = 25
' Draw the Analog Graph
For index As Integer = 0 To binArray.Length - 1
If binArray(index) = 0 Then
Sin0(xPos, yPos)
xPos += 40
Else
Sin1(xPos, yPos)
xPos += 40
End If
Next
' Clean up memory
objThinPen.Dispose()
objThickPen.Dispose()
objFont.Dispose()
g.Dispose()
End Sub
'=========================================================================================================================================
Private Sub clearGraph()
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Clear the Graph
g.Clear(System.Drawing.SystemColors.ButtonFace)
'Clean up memory
g.Dispose()
End Sub
'=========================================================================================================================================
' Sine wave for 0
Private Sub Sin0(ByVal x As Integer, ByVal y As Integer)
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create Pen
Dim objThickPen = New Pen(Drawing.Color.Blue, 2)
' Set up points to draw the top half of the first sine graph
Dim pt1 = New Point(x, y)
Dim pt2 = New Point(x + 5, y - 77)
Dim pt3 = New Point(x + 10, y)
' Draw the top half of the first sine wave
g.DrawBezier(objThickPen, pt1, pt1, pt2, pt3)
' Set up points to draw the lower half of the first sine graph
pt1 = New Point(x + 10, y)
pt2 = New Point(x + 15, y + 77)
pt3 = New Point(x + 20, y)
' Draw the lower half of the first sine wave
g.DrawBezier(objThickPen, pt1, pt1, pt2, pt3)
''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''
' Set up points to draw the top half of the second sine graph
pt1 = New Point(x + 20, y)
pt2 = New Point(x + 25, y - 77)
pt3 = New Point(x + 30, y)
' Draw the top half of the second sine wave
g.DrawBezier(objThickPen, pt1, pt1, pt2, pt3)
' Set up points to draw the lower half of the second sine graph
pt1 = New Point(x + 30, y)
pt2 = New Point(x + 35, y + 77)
pt3 = New Point(x + 40, y)
' Draw the lower half of the second sine wave
g.DrawBezier(objThickPen, pt1, pt1, pt2, pt3)
'Clean up memory
objThickPen.Dispose()
g.Dispose()
End Sub
'=========================================================================================================================================
' Sine wave for 1
Private Sub Sin1(ByVal x As Integer, ByVal y As Integer)
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create Pen
Dim objThickPen = New Pen(Drawing.Color.Blue, 2)
' Set up points to draw the top half of the sine graph
Dim pt1 = New Point(x, y)
Dim pt2 = New Point(x + 10, y - 77)
Dim pt3 = New Point(x + 20, y)
' Draw the top half of the sine wave
g.DrawBezier(objThickPen, pt1, pt1, pt2, pt3)
' Set up points to draw the lower half of the sine graph
pt1 = New Point(x + 20, y)
pt2 = New Point(x + 30, y + 77)
pt3 = New Point(x + 40, y)
' Draw the lower half of the sine wave
g.DrawBezier(objThickPen, pt1, pt1, pt2, pt3)
'Clean up memory
objThickPen.Dispose()
g.Dispose()
End Sub
'=========================================================================================================================================
' Line for 0 -> 0
Private Sub Line1(ByVal x As Integer, ByVal y As Integer)
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create Pen
Dim objThickPen = New Pen(Drawing.Color.Blue, 3)
' Draw the line
g.DrawLine(objThickPen, x, y, x + 20, y)
'Clean up memory
objThickPen.Dispose()
g.Dispose()
End Sub
'=========================================================================================================================================
' Line for 0 -> 1
Private Sub Line2(ByVal x As Integer, ByVal y As Integer)
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create Pen
Dim objThickPen = New Pen(Drawing.Color.Blue, 3)
' Draw the line
g.DrawLine(objThickPen, x, y, x + 20, y)
g.DrawLine(objThickPen, x + 20, y, x + 20, y - 20)
'Clean up memory
objThickPen.Dispose()
g.Dispose()
End Sub
'=========================================================================================================================================
' Line for 1 -> 1
Private Sub Line3(ByVal x As Integer, ByVal y As Integer)
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create Pen
Dim objThickPen = New Pen(Drawing.Color.Blue, 3)
' Draw the line
g.DrawLine(objThickPen, x, y - 20, x + 20, y - 20)
'Clean up memory
objThickPen.Dispose()
g.Dispose()
End Sub
'=========================================================================================================================================
' Line for 1 -> 0
Private Sub Line4(ByVal x As Integer, ByVal y As Integer)
' Create graphics
Dim g As Graphics
g = picGraph.CreateGraphics
' Create Pen
Dim objThickPen = New Pen(Drawing.Color.Blue, 3)
' Draw the line
g.DrawLine(objThickPen, x, y - 20, x + 20, y - 20)
g.DrawLine(objThickPen, x + 20, y - 20, x + 20, y)
'Clean up memory
objThickPen.Dispose()
g.Dispose()
End Sub
End Class