jwcoleman87
Well-known member
- Joined
- Oct 4, 2014
- Messages
- 124
- Programming Experience
- Beginner
I have this form which is designed to be a home page of sorts for other forms and modules. The trick is that the user logging in to this home page will have access to modules based off of what they are given as defined in the database. So, depending on what kind of access they have they need buttons to be able to open the different modules (other forms n such). I have devised a way to do this by using a flow layout panel and a bunch of dummy buttons (24 to be exact). All of these buttons are invisible until set visible when the text for the button is changed to the name of a corresponding module. Every single button connects to a single event handler that compares the button text to the name of a module, and executes it. It's like this:
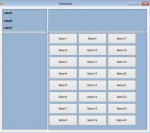
And the code looks like this:
I guess my question to the general discussion of vbdotnetforums is simple, are there more efficient ways of accomplishing this task?
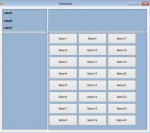
And the code looks like this:
Public Class LandingZone Dim User As String Dim Workstation As String Dim Position As String 'Need to grab a list of all modules, need to match modules with name Private Sub LandingZone_Load(sender As Object, e As EventArgs) Handles MyBase.Load Using db As New ProductionDataModelDataContext Dim loginform As New Login() If loginform.ShowDialog(Me) = Windows.Forms.DialogResult.OK Then Me.User = loginform.txtUserName.Text Me.lblName.Text = Me.User Dim job = From uj In db.TTUserJobs _ Join u In db.TTUsers On uj.UserID Equals u.TTUserID _ Join j In db.TTJobs On uj.JobID Equals j.JobID _ Where u.TTUserID = Me.User AndAlso j.JobID = uj.JobID _ Select j.JobTitle Me.Position = job.First Me.lblPosition.Text = Me.Position ShowAvailableModules() lblWorkstation.Text = Environment.MachineName Me.Show() Me.Enabled = True End If End Using End Sub Private Sub MatchButtonWithModule(buttonText As String) 'IF the BUTTON TEXT is blahblahblah THEN If buttonText = "Repair" Then Dim TechProductionTracker As New TechInterface(Me.User, Me.Position) TechProductionTracker.Show() Me.Visible = False ElseIf buttonText = "Lead Production Tracker" Then LeadInterface.Show() Me.Visible = False Else MsgBox("Module not yet supported.") End If 'DO SOME STUFF 'ELSE IF the button name is derpderpderp THEN 'DO SOME OTHER STUFF 'DO THIS CRAP OVER AND OVER FOR EACH MODULE End Sub Private Sub ShowAvailableModules() Using db As New ProductionDataModelDataContext 'for this magic to happen, accessiblemodules must be created within this function Dim AccessibleModules = From m In db.TTModules _ Join jm In db.TTJobModules On m.ModuleID Equals jm.ModuleID _ Join j In db.TTJobs On jm.JobTitle Equals j.JobTitle _ Join uj In db.TTUserJobs On j.JobID Equals uj.JobID _ Join u In db.TTUsers On uj.UserID Equals u.TTUserID _ Where u.TTUserID = Me.User _ Select m.ModuleName Dim index As Integer = 0 Do While index < AccessibleModules.ToList().Count FlowLayoutPanel1.Controls.Item(index).Text = AccessibleModules(index) FlowLayoutPanel1.Controls.Item(index).Visible = True index += 1 Loop 'FOR AS MANY MODULES AVAILABLE LOOP THROUGH buttons in FLOWLAYOUTPANEL 'SET BUTTON TEXT to INDEX OF MODULE NAME 'SET BUTTON TO VISIBLE End Using End Sub Private Sub ButtonMagic(sender As Object, e As EventArgs) Handles Button1.Click, Button2.Click, Button3.Click, Button4.Click, _ Button5.Click, Button6.Click, Button7.Click, Button8.Click, _ Button9.Click, Button10.Click, Button11.Click, Button12.Click, _ Button13.Click, Button14.Click, Button15.Click, Button16.Click, _ Button17.Click, Button18.Click, Button19.Click, Button20.Click, _ Button21.Click, Button22.Click, Button23.Click, Button24.Click MatchButtonWithModule(sender.text) End Sub End Class
I guess my question to the general discussion of vbdotnetforums is simple, are there more efficient ways of accomplishing this task?