jwcoleman87
Well-known member
- Joined
- Oct 4, 2014
- Messages
- 124
- Programming Experience
- Beginner
I will try to make this post as coherent as possible while the intention of this post is to gather my thoughts and get myself going in the right direction, thus possibly leading to some incoherence. That's where you guys come in! :distant:
What I'm trying to do is add and remove tab pages on the fly. These tab pages will contain controls which have handlers for them. I'm expecting to have to predetermine some of these things before run time, such as the handlers and parameters, some things can be done on the fly, such as the organization of the controls (using flow layout panels). This all looks something like this:
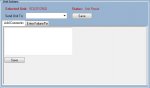
The code to create these tab pages looks like this:
And:
As you can see, they have some things in common, the difference lies in the input methods and controls used to gather that input. I am presuming that I will have to hard code most of this. What I'm wondering about is a way to abstract these. I was thinking I could make a base class that had an overridable constructor in them. If I did this then when I went to design a new action tab I could simply derive it from the base class and carry over the common things. I'm currently trying to determine what the common things really are.
A going list I currently have running around my head:
These I believe cover the standardized things, the basic layout of each tab page. What is seemingly more complex to me is the abstraction of the stuff that lies within. There are lots of definitive indefinite variables, such as: (please focus your expertise and responses in this area)
Other things????
Please help! I expect this to be a pretty long and drawn out thread.
What I'm trying to do is add and remove tab pages on the fly. These tab pages will contain controls which have handlers for them. I'm expecting to have to predetermine some of these things before run time, such as the handlers and parameters, some things can be done on the fly, such as the organization of the controls (using flow layout panels). This all looks something like this:
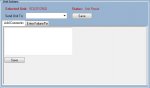
The code to create these tab pages looks like this:
'This creates the AddComments Tab Dim CommentsTab As New TabPage With {.Name = "Comments", .Text = "Add Comments"} TabControl1.TabPages.Add(CommentsTab) Dim flpcomments As New FlowLayoutPanel With {.Parent = TabControl1.TabPages("Comments"), .Dock = DockStyle.Fill, .BorderStyle = BorderStyle.Fixed3D, .FlowDirection = FlowDirection.TopDown} Dim txtComments As New TextBox With {.Parent = flpcomments, .Name = "CommentBox", .Width = 250, .Height = 100, .Multiline = True} Dim btnSaveComment As New Button With {.Parent = flpcomments, .Text = "Save"} AddHandler btnSaveComment.Click, AddressOf Me.SaveComment Dim rc As New RepairControl
And:
'This creates the enter Failure/Fix Tab Dim FailureFixTab As New TabPage With {.Name = "FailureFix", .Text = "Enter Failure/Fix"} TabControl1.TabPages.Add(FailureFixTab) Dim flpFailureFix As New FlowLayoutPanel With {.Parent = TabControl1.TabPages("FailureFix"), .Dock = DockStyle.Fill, .BorderStyle = BorderStyle.Fixed3D, .FlowDirection = FlowDirection.TopDown} Dim cbSelectedFailure As New ComboBox With {.Parent = flpFailureFix, .DataSource = rc.GetFailureFix(1), .SelectedItem = Nothing} Dim cbSelectedFix As New ComboBox With {.Parent = flpFailureFix, .DataSource = rc.GetFailureFix(2), .SelectedItem = Nothing} Dim btnSaveFailureFix As New Button With {.Parent = flpFailureFix, .Text = "Save"} AddHandler btnSaveFailureFix.Click, AddressOf Me.SaveFailureFix
As you can see, they have some things in common, the difference lies in the input methods and controls used to gather that input. I am presuming that I will have to hard code most of this. What I'm wondering about is a way to abstract these. I was thinking I could make a base class that had an overridable constructor in them. If I did this then when I went to design a new action tab I could simply derive it from the base class and carry over the common things. I'm currently trying to determine what the common things really are.
A going list I currently have running around my head:
- Tab Control (parent of the tab page)
- Tab name
- Tab text
- FlowlayoutPanel name
- Flowlayoutpanel Parent (The tab page we created)
- FlowLayoutPanel Dock
- FlowLayoutPanel Flowdirection
These I believe cover the standardized things, the basic layout of each tab page. What is seemingly more complex to me is the abstraction of the stuff that lies within. There are lots of definitive indefinite variables, such as: (please focus your expertise and responses in this area)
- An indefinite number of controls (perhaps this could be abstracted as a "control collection"?)
- An indefinite number of handlers for these controls (perhaps a param array of some sort?)
- An indefinite number of parameters for the methods called by the events (this is based on the control collection I think?)
Other things????
Please help! I expect this to be a pretty long and drawn out thread.