I have built a form which is used to input sales expenses. It consists of a few text boxes (for Expense Header information) and the DevExpress DataGrid (for Expense Details entries). The Textboxes are bound to the ExpenseHdr table in the ExpenseTickets Dataset and the grid is bound to a table called ExpenseLines in the ExpenseTickets Dataset.
When I load the form, I am able to pass in an existing ExpenseID parameter to load an existing ticket or I can pass in 0 which will instruct the form to generate a new ExpenseID and create a new Ticket.
When I pass in 0, I generate a new ExpenseID by calling a stored procedure that will return the next available ExpenseID. I then run my select statement with the new ExpenseID against the ExpenseHdr table. This will of course retrieve 0 rows and give me an empty Table inside the Dataset. I then bind the TextBoxes to the ExpenseHdr Table. I then automatically populate some information in the text boxes (like the ExpenseID etc.)
This is where the problem comes in. When I run the save procedure, I do not receive any errors nor does it save the data to the SQL database.
I have written the code myself to perform this function and I think I know what the issue is. When I auto populate the text boxes, I have not instructed the dataset/table to create a new record/row. However I added code to do that and it still did not fix the problem. Does this have anything to do with not using a binding source? The form will only ever have 1 record in it at anyone 1 time so I do not need to navigate multiple records. The grid will have multiple records loaded but it handles it's own binding.
Here is the code relevant to the ExpenseHeader, can anybody help me figure this out?
Thanks
I have also attached a screen shot of the form.
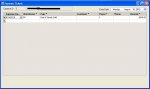
When I load the form, I am able to pass in an existing ExpenseID parameter to load an existing ticket or I can pass in 0 which will instruct the form to generate a new ExpenseID and create a new Ticket.
When I pass in 0, I generate a new ExpenseID by calling a stored procedure that will return the next available ExpenseID. I then run my select statement with the new ExpenseID against the ExpenseHdr table. This will of course retrieve 0 rows and give me an empty Table inside the Dataset. I then bind the TextBoxes to the ExpenseHdr Table. I then automatically populate some information in the text boxes (like the ExpenseID etc.)
This is where the problem comes in. When I run the save procedure, I do not receive any errors nor does it save the data to the SQL database.
I have written the code myself to perform this function and I think I know what the issue is. When I auto populate the text boxes, I have not instructed the dataset/table to create a new record/row. However I added code to do that and it still did not fix the problem. Does this have anything to do with not using a binding source? The form will only ever have 1 record in it at anyone 1 time so I do not need to navigate multiple records. The grid will have multiple records loaded but it handles it's own binding.
Here is the code relevant to the ExpenseHeader, can anybody help me figure this out?
Thanks
VB.NET:
Imports System.Data
Imports System.Data.SqlClient
Public Class ExpenseTickets
'Connections
Dim PhantomConn As SqlConnection
'DataSet
Dim ExpenseTicketsDataSet As DataSet
'ExpenseHeader
Dim ExpenseHdrAdapter As New SqlDataAdapter
Dim ExpenseHdrCommandBuilder As SqlCommandBuilder
Private Sub ExpenseTickets_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
'set connection string
PhantomConn = New SqlConnection(My.Settings.PhantomConnectionString)
If LoadExpenseID = 0 Then
'we are generating a new ExpenseTicket. Get a new ExpenseID
LoadExpenseID = GetExpenseIDKey()
'check if LoadExpenseID now have a value
If LoadExpenseID = 0 Then
MsgBox("Expense ID cannot be 0, the form will now close. Please report this error to the I.T. Department", MsgBoxStyle.Critical)
CloseFrmDuringLoad(Me)
Exit Sub
End If
End If
'load the expense Header DataSet
LoadExpenseHdr()
'if we are adding a new record, auto fill some fields.
If EditMode = "A" Then
ExpenseTicketsDataSet.Tables("ExpenseHdr").NewRow()
ExpenseID.Text = LoadExpenseID
ExpenseUserID.Text = GetExpenseUserID(CurrentUser.EmployeeID)
ExpenseStatus.Text = "E"
EntryDate.Value = Date.Today
End If
End Sub
Private Function GetExpenseIDKey() As Integer
'This will be used to generate the Expense Type Key.
Dim SQLComm As SqlCommand
SQLComm = New SqlCommand("GetKeySp", PhantomConn)
SQLComm.CommandType = CommandType.StoredProcedure
'4 is the ID for the ExpenseID Key.
SQLComm.Parameters.Add(New SqlParameter("@KeyID", 4))
SQLComm.Parameters.Add("@Return", SqlDbType.Int)
SQLComm.Parameters("@Return").Direction = ParameterDirection.ReturnValue
Try
PhantomConn.Open()
SQLComm.ExecuteNonQuery()
Return SQLComm.Parameters("@Return").Value
Catch ex As Exception
MsgBox(ex.Message, MsgBoxStyle.Critical)
Return SQLComm.Parameters("@Return").Value
Finally
PhantomConn.Close()
End Try
End Function
Private Sub LoadExpenseHdr()
ExpenseHdrAdapter.SelectCommand = New SqlCommand
ExpenseHdrAdapter.SelectCommand.Connection = PhantomConn
ExpenseHdrAdapter.SelectCommand.CommandText = "Select * From ExpenseHdr Where ExpenseID = " & LoadExpenseID
'automatically create insert/delete/update statements
ExpenseHdrCommandBuilder = New SqlCommandBuilder(ExpenseHdrAdapter)
ExpenseTicketsDataSet = New DataSet()
ExpenseHdrAdapter.Fill(ExpenseTicketsDataSet, "ExpenseHdr")
'bind text boxes to the dataset.
ExpenseID.DataBindings.Add("Text", ExpenseTicketsDataSet, "ExpenseHdr.ExpenseID")
ExpenseUserID.DataBindings.Add("Text", ExpenseTicketsDataSet, "ExpenseHdr.ExpenseUserID")
ExpenseStatus.DataBindings.Add("Text", ExpenseTicketsDataSet, "ExpenseHdr.ExpenseStatus")
EntryDate.DataBindings.Add("Value", ExpenseTicketsDataSet, "ExpenseHdr.EntryDate")
End Sub
Public Function SaveRecord() As Boolean Implements PublicFunctions.IRecordEditor.SaveRecord
If Me.Validate() = True Then
'run the save in a try catch statement
Try
'save the Expense Header record
ExpenseHdrAdapter.Update(ExpenseTicketsDataSet, "ExpenseHdr")
Catch Ex As Exception
DataError = True
MsgBox(Ex.Message, MsgBoxStyle.Critical)
End Try
If DataError = False Then
MsgBox("Save Complete")
'flag the save function as true
Return True
End If
Else
MsgBox("Cannot save, please resolve any validation errors before saving.", MsgBoxStyle.Exclamation)
Return False
End If
End Function
End Class
I have also attached a screen shot of the form.
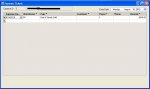