It is as far as I know not possible to detect this directly from the process or by other means. One possible approach I can think of is to enumerate and analyze the windows using native Windows Api. Windows is a lot more than one usually thinks, combination of styles and features make up the application windows you usually see, which in short usually are visible toplevel windows that have a main window title (there are some exceptions and more to it). I have something of a tool application that uses the EnumWindows api to look into these windows and check/filter by window styles, the project is attached and here is a screenshot:
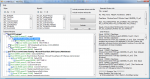
What I found is that an unhandled exception window is shown as a dialog, which is also a common window style, dialogs usually have the exstyle DLGMODALFRAME flag set. To set those apart I found that the exception window have the APPWINDOW exstyle set, which regular dialogs usually don't. You can give the application a spin in your environment and see how reliably it detects those windows, perhaps are there other criteria you need to use. For example you could be targeting specific processes that make the detection simpler to not interfere with other regular operations.
The attached project give insight and code samples to using the EnumWindows and related Api functions.
Documentation for windows styles are given here
Window Styles (Windows) and here
Extended Window Styles (Windows).
An introduction to windows from Api point of view is here:
Window Overviews (Windows).
To sum it up, if this approach prove to achieve the goal then your implementation will be a lot simpler than the attached project. Given the conditions you need a call to EnumWindows, in callback get title and styles, check if there is a title and if exstyle has flags APPWINDOW and DLGMODALFRAME. If you use the WindowInfo class and the NativeMethods module this will comprise to these few lines of code:
Private Sub FindExceptions()
EnumWindows(New EnumWindowsProc(AddressOf Callback), IntPtr.Zero)
End Sub
Private Function Callback(ByVal hwnd As IntPtr, ByVal lParam As IntPtr) As Boolean
Dim info As New WindowInfo(hwnd)
If info.Title <> String.Empty AndAlso info.StyleEx.HasStyleEx(WindowStyleEx.WS_EX_APPWINDOW Or WindowStyleEx.WS_EX_DLGMODALFRAME) Then
Debug.WriteLine(String.Format("Appears to have crashed: pid {0} : {1}", info.Pid, info.Title))
' if this is true:
'Process.GetProcessById(info.Pid).Kill()
End If
Return True
End Function
There is one more thing that can be done, using EnumChildWindows you can list the control windows that belong to a toplevel window and also get the text from these. So when you have found a dialog window you could also do a text search to see if the dialog expose for example "Unhandled exception". The project also include this, you may be able to see that text in the screenshot.
I see your Framework is .NET 2.0, the project is .Net 4.0 and uses at least one feature from it, this can be worked around or you could finally upgrade to VB 2010
