R0gueHunt3R
New member
- Joined
- Mar 28, 2011
- Messages
- 4
- Programming Experience
- Beginner
As part of an assignment, I need to add numbers which are displayed in a rich text box. The numbers are read from a text box. The user needs to be able to input the numbers as many times as possible. Since, the numbers all come from the same text box, I am unsure how to retain the old information and add the new information to it.
Since I am not really able to make my point clear, I attach this picture:
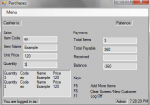
As you can see, I also have a problem with the heading repeating itself. Is there a way of forcing it to only appear the first time?
Here is the code:
Since I am not really able to make my point clear, I attach this picture:
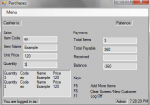
As you can see, I also have a problem with the heading repeating itself. Is there a way of forcing it to only appear the first time?
Here is the code:
VB.NET:
Private Sub AddMoreItemsToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles AddMoreItemsToolStripMenuItem.Click
'Retrieve number from textboxes
intUnitPrice = Val(UnitPrice_txt.Text)
intQuantity = Val(Quantity_txt.Text)
intTotal = Val(Total_txt.Text)
intReceived = Val(Received_txt.Text)
'Display the output header
ItemBox_txt.Text &= ("Quantity") & vbTab & ("Code") & vbTab & ("Name") & vbTab & ("Price") & Environment.NewLine
'Display the Data
ItemBox_txt.Text &= (intQuantity) & vbTab & (ItemCode_txt.Text) & vbTab & (ItemName_txt.Text) & vbTab & (intUnitPrice) & Environment.NewLine
'Calculations
intTotal = intUnitPrice * intQuantity
intBalance = intReceived - intTotal
'Display result in label
TotalItems_txt.Text = intQuantity
Total_txt.Text = intTotal
Balance_txt.Text = intBalance
End Sub